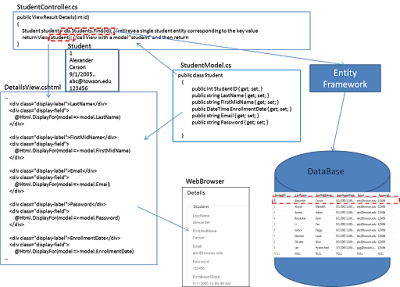
Labels
Programming
(16)
Algorithm
(15)
Java
(15)
ASP .NET
(4)
Enterprise Architecture
(4)
PHP and MySQL
(4)
Software Engineering
(2)
C#
(1)
Data Mining
(1)
Deep Learning
(1)
Java Script
(1)
Python
(1)
Friday, June 17, 2011
ASP .NET MVC3 Application Structure with Code(Read Data))
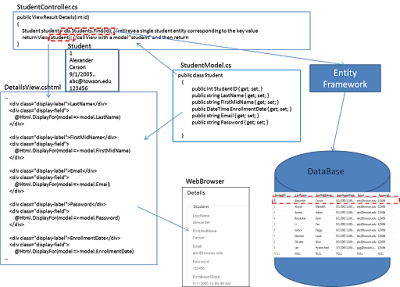
Saturday, June 11, 2011
Web Application MVC Project Structure in ASP .NET
ASP .NET MVC3 ViewBag
A ViewBag is a dynamic object that can be used to pass data from Controllers to Views as you use ViewData[]. It enables developers can use get/set values and add additional fields without strongly-typed classes. To understand this concepts, let's compare ViewData[] and ViewBag.
- Using ViewData[]
-Controller
public ActionResult Index()
{
List<string> colors = new List<string>();
colors.Add("red");
colors.Add("green");
colors.Add("blue");
ViewData["listColors"] = colors;
ViewData["dateNow"] = DateTime.Now;
ViewData["name"] = "Hajan";
ViewData["age"] = 25;
return View();
}
{
List<string> colors = new List<string>();
colors.Add("red");
colors.Add("green");
colors.Add("blue");
ViewData["listColors"] = colors;
ViewData["dateNow"] = DateTime.Now;
ViewData["name"] = "Hajan";
ViewData["age"] = 25;
return View();
}
-Views
<p>
My name is
<b><%: ViewData["name"] %>b>,
<b><%: ViewData["age"] %>b> years old.
<br />
I like the following colors:
p>
<ul id="colors">
<% foreach (var color in ViewData["listColors"] as List<string>){ %>
<li>
<font color="<%: color %>"><%: color %>font>
li>
<% } %>
ul>
<p>
<%: ViewData["dateNow"] %>
p>
My name is
<b><%: ViewData["name"] %>b>,
<b><%: ViewData["age"] %>b> years old.
<br />
I like the following colors:
p>
<ul id="colors">
<% foreach (var color in ViewData["listColors"] as List<string>){ %>
<li>
<font color="<%: color %>"><%: color %>font>
li>
<% } %>
ul>
<p>
<%: ViewData["dateNow"] %>
p>
- Using ViewBag
-Controller
public ActionResult Index()
{
List<string> colors = new List<string>();
colors.Add("red");
colors.Add("green");
colors.Add("blue");
ViewBag.ListColors = colors; //colors is List
ViewBag.DateNow = DateTime.Now;
ViewBag.Name = "Hajan";
ViewBag.Age = 25;
return View();
}
{
List<string> colors = new List<string>();
colors.Add("red");
colors.Add("green");
colors.Add("blue");
ViewBag.ListColors = colors; //colors is List
ViewBag.DateNow = DateTime.Now;
ViewBag.Name = "Hajan";
ViewBag.Age = 25;
return View();
}
-Views
<p>
My name is
<b><%: ViewBag.Name %>b>,
<b><%: ViewBag.Age %>b> years old.
<br />
I like the following colors:
p>
<ul id="colors">
<% foreach (var color in ViewBag.ListColors) { %>
<li>
<font color="<%: color %>"><%: color %>font>
li>
<% } %>
ul>
<p>
<%: ViewBag.DateNow %>
p>
My name is
<b><%: ViewBag.Name %>b>,
<b><%: ViewBag.Age %>b> years old.
<br />
I like the following colors:
p>
<ul id="colors">
<% foreach (var color in ViewBag.ListColors) { %>
<li>
<font color="<%: color %>"><%: color %>font>
li>
<% } %>
ul>
<p>
<%: ViewBag.DateNow %>
p>
Change the body style in ASP .NET framework
Subscribe to:
Posts (Atom)